Cosmological Source Catalog¶
SOXS provides the make_cosmological_sources_file()
function to generate a set of photons from cosmological halos and store them in a SIMPUT catalog.
First, import our modules:
[1]:
import matplotlib
matplotlib.rc("font", size=18)
import soxs
Second, define our parameters, including the location within the catalog where we are pointed via the argument cat_center
. To aid in picking a location, see the halo map.
[2]:
exp_time = (200.0, "ks")
fov = 20.0 # in arcmin
sky_center = [30.0, 45.0] # in degrees
cat_center = [3.1, -1.9]
Now, use make_cosmological_sources_file()
to create a SIMPUT catalog made up of photons from the halos. We’ll set a random seed using the prng
parameter to make sure we get the same result every time. We will also write the halo properties to an ASCII table for later analysis, using the output_sources
parameter:
[3]:
soxs.make_cosmological_sources_file(
"my_cat.simput",
"cosmo",
exp_time,
fov,
sky_center,
cat_center=cat_center,
prng=33,
overwrite=True,
)
soxs : [INFO ] 2024-06-19 15:58:48,483 Creating photons from cosmological sources.
soxs : [INFO ] 2024-06-19 15:58:48,486 Loading halo data from catalog: /Users/jzuhone/Source/soxs/soxs/files/halo_catalog.h5
soxs : [INFO ] 2024-06-19 15:58:48,584 Coordinates of the FOV within the catalog are (3.1, -1.9) deg.
soxs : [INFO ] 2024-06-19 15:58:48,584 Selecting halos in the FOV.
soxs : [INFO ] 2024-06-19 15:58:48,589 Number of halos in the field of view: 384
soxs : [INFO ] 2024-06-19 15:58:58,531 Created 5750617 photons from cosmological sources.
soxs : [INFO ] 2024-06-19 15:58:58,614 Appending source 'cosmo' to my_cat.simput.
[3]:
<soxs.simput.SimputCatalog at 0x11fcaec00>
Next, use the instrument_simulator()
to simulate the observation:
[4]:
soxs.instrument_simulator(
"my_cat.simput",
"cosmo_cat_evt.fits",
exp_time,
"lynx_hdxi",
sky_center,
overwrite=True,
)
soxs : [INFO ] 2024-06-19 15:58:58,703 Making observation of source in cosmo_cat_evt.fits.
soxs : [INFO ] 2024-06-19 15:58:58,877 Detecting events from source cosmo.
soxs : [INFO ] 2024-06-19 15:58:58,878 Applying energy-dependent effective area from xrs_hdxi_3x10.arf.
soxs : [INFO ] 2024-06-19 15:58:59,063 Pixeling events.
soxs : [INFO ] 2024-06-19 15:58:59,380 Scattering events with a image-based PSF.
soxs : [INFO ] 2024-06-19 15:58:59,658 2156617 events were detected from the source.
soxs : [INFO ] 2024-06-19 15:58:59,772 Scattering energies with RMF xrs_hdxi.rmf.
soxs : [INFO ] 2024-06-19 15:59:02,488 Adding background events.
soxs : [INFO ] 2024-06-19 15:59:02,558 Adding in point-source background.
soxs : [INFO ] 2024-06-19 15:59:12,637 Detecting events from source ptsrc_bkgnd.
soxs : [INFO ] 2024-06-19 15:59:12,638 Applying energy-dependent effective area from xrs_hdxi_3x10.arf.
soxs : [INFO ] 2024-06-19 15:59:14,065 Pixeling events.
soxs : [INFO ] 2024-06-19 15:59:15,046 Scattering events with a image-based PSF.
soxs : [INFO ] 2024-06-19 15:59:15,830 6810956 events were detected from the source.
soxs : [INFO ] 2024-06-19 15:59:16,077 Scattering energies with RMF xrs_hdxi.rmf.
soxs : [INFO ] 2024-06-19 15:59:23,482 Generated 6810956 photons from the point-source background.
soxs : [INFO ] 2024-06-19 15:59:23,482 Adding in astrophysical foreground.
soxs : [INFO ] 2024-06-19 15:59:34,745 Adding in instrumental background.
soxs : [INFO ] 2024-06-19 15:59:36,303 Making 34168488 events from the galactic foreground.
soxs : [INFO ] 2024-06-19 15:59:36,305 Making 524136 events from the instrumental background.
soxs : [INFO ] 2024-06-19 15:59:40,817 Writing events to file cosmo_cat_evt.fits.
soxs : [INFO ] 2024-06-19 15:59:47,081 Observation complete.
We can use the write_image()
function in SOXS to bin the events into an image and write them to a file, restricting the energies between 0.7 and 7.0 keV:
[5]:
soxs.write_image(
"cosmo_cat_evt.fits", "cosmo_img.fits", emin=0.7, emax=7.0, overwrite=True
)
We can now show the resulting image:
[6]:
fig, ax = soxs.plot_image(
"cosmo_img.fits",
stretch="sqrt",
cmap="cubehelix",
vmin=0.0,
vmax=2.0,
width=0.33333,
)
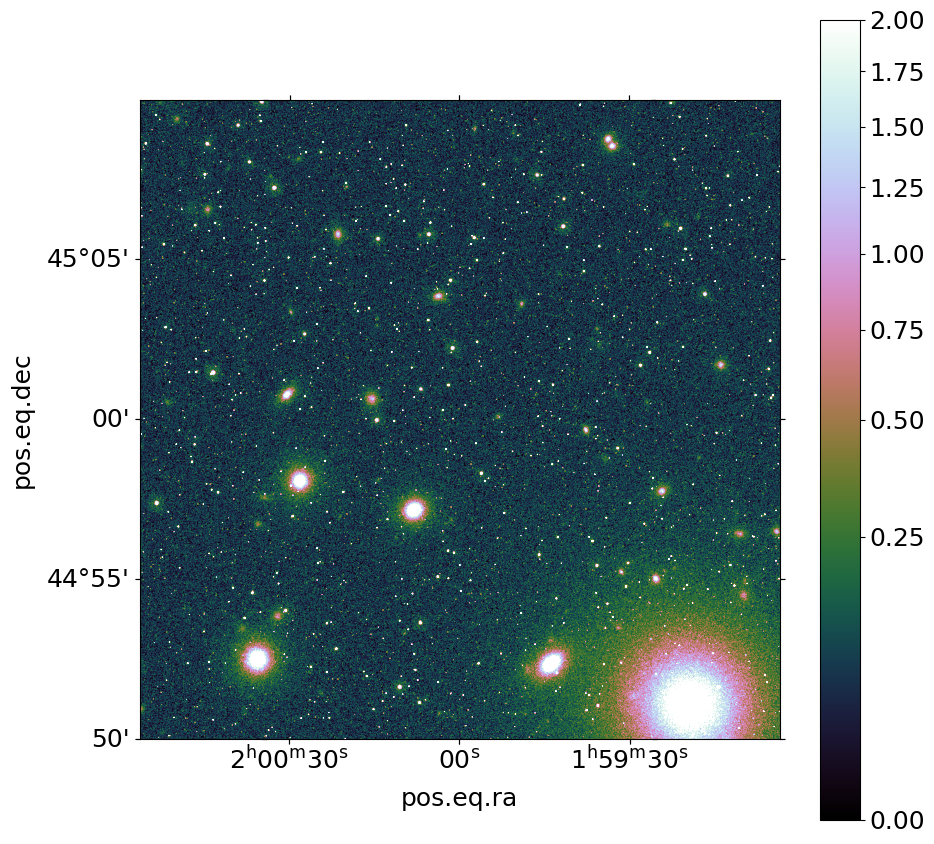