Point Source Catalog¶
Though SOXS creates events from point sources as part of the background, one may want to study the point source properties in detail, and desire finer-grained control over their generation. SOXS provides the make_point_sources_file()
function for this purpose, to create a set of photons from point sources using the point-source background model and store them in a SIMPUT catalog.
First, import our modules:
[1]:
import matplotlib
matplotlib.rc("font", size=18)
import soxs
Second, define our parameters:
[2]:
exp_time = (300.0, "ks") # in seconds
fov = 20.0 # in arcmin
sky_center = [22.0, -27.0] # in degrees
Now, use make_point_sources_file()
to create a SIMPUT catalog made up of photons from point sources. We’ll set a random seed using the prng
parameter to make sure we get the same result every time. We will also write the point source properties to an ASCII table for later analysis, using the output_sources
parameter:
[3]:
soxs.make_point_sources_file(
"my_cat.simput",
"ptsrc",
exp_time,
fov,
sky_center,
prng=24,
output_sources="point_source_table.dat",
overwrite=True,
)
soxs : [INFO ] 2025-02-18 14:00:40,077 Appending source 'ptsrc' to my_cat.simput.
[3]:
<soxs.simput.SimputCatalog at 0x1232170e0>
In a subsequent invocation of make_point_sources_file()
, one could use the ASCII table of sources as an input to generate events from the same sources, using the input_sources
keyword argument.
Next, use the instrument_simulator()
to simulate the observation. Since we explicitly created a SIMPUT catalog of point sources, we should turn the automatic point-source background in SOXS off by setting ptsrc_bkgnd=False
:
[4]:
soxs.instrument_simulator(
"my_cat.simput",
"ptsrc_cat_evt.fits",
exp_time,
"lynx_hdxi",
sky_center,
overwrite=True,
ptsrc_bkgnd=False,
)
soxs : [INFO ] 2025-02-18 14:00:40,361 Simulating events from 1 sources using instrument lynx_hdxi for 300 ks.
soxs : [INFO ] 2025-02-18 14:00:43,432 Scattering energies with RMF xrs_hdxi.rmf.
soxs : [INFO ] 2025-02-18 14:00:49,775 Detected 5576568 events in total.
soxs : [INFO ] 2025-02-18 14:00:49,798 Adding background events.
soxs : [INFO ] 2025-02-18 14:00:49,860 Adding in astrophysical foreground.
soxs : [INFO ] 2025-02-18 14:01:00,183 Adding in instrumental background.
soxs : [INFO ] 2025-02-18 14:01:02,655 Making 50601017 events from the galactic foreground.
soxs : [INFO ] 2025-02-18 14:01:02,658 Making 784584 events from the instrumental background.
soxs : [INFO ] 2025-02-18 14:01:25,808 Observation complete.
soxs : [INFO ] 2025-02-18 14:01:25,826 Writing events to file ptsrc_cat_evt.fits.
We can use the write_image()
function in SOXS to bin the events into an image and write them to a file, restricting the energies between 0.7 and 2.0 keV:
[5]:
soxs.write_image(
"ptsrc_cat_evt.fits", "ptsrc_img.fits", emin=0.7, emax=2.0, overwrite=True
)
We can now show the resulting image:
[6]:
fig, ax = soxs.plot_image(
"ptsrc_img.fits",
stretch="sqrt",
cmap="plasma",
width=0.1,
vmin=0.0,
vmax=1.0,
center=[22.0, -27.0],
)
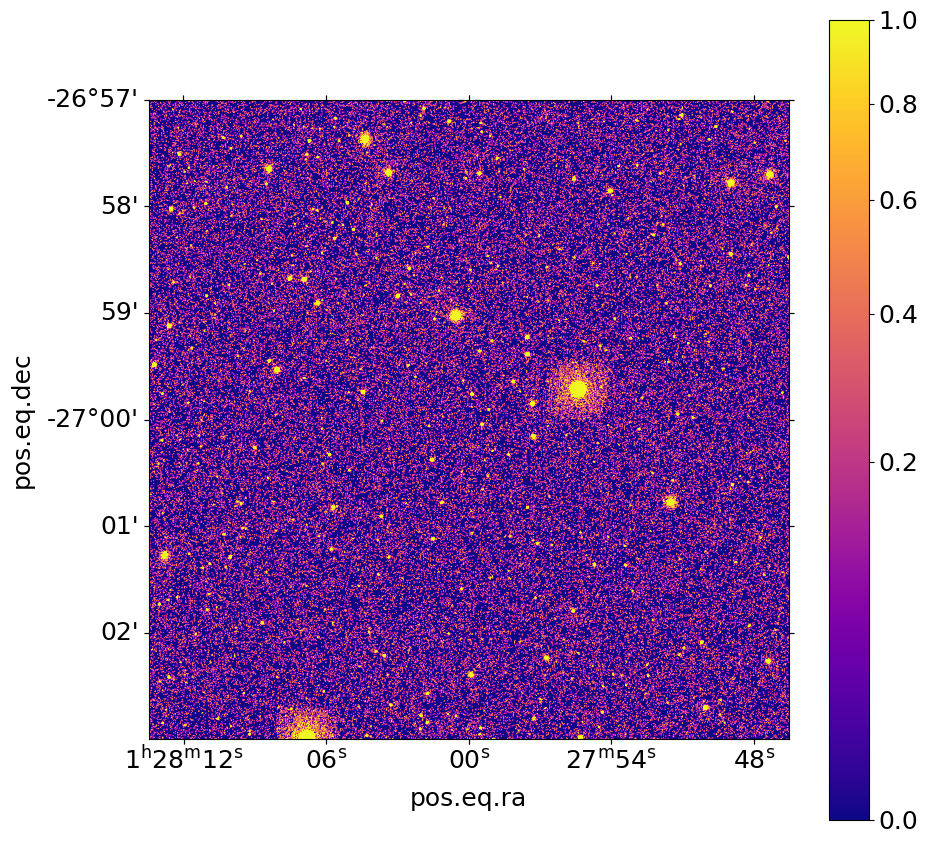